It is fitting that my first post on this site is about custom menus with Google Apps Script, because, often, custom menus are your users’ first touch with your custom functionality. Your users will be surprised the first time they see the custom menu on their Google App UI. And they will be delighted when the functionality fulfills their expectations. This post will show how to surprise and delight your users with the Google Apps Script UI menus.

How Custom Menus Work
Custom menus allow you to execute the Google Apps Script functions that you have defined. Each menu item, when clicked, triggers the Google Apps Script function that corresponds to that menu item. Custom menus are easy to create, and we will get to that in a minute, but let’s get a few questions out of the way first. Menus can be created and modified*, but menus cannot be deleted. Most often, Menus are created with the onOpen()
function, but they can also be created by an ay trigger or event. For example, you could use a menu item click to create another menu. Similarly, many Google Docs add-ons use the add-on menu to create a menu to offer access to the functions of their add-on. Unfortunately, Menus cannot be dynamically created. The menu item names can be, but the functions that the menu items trigger cannot be. This will become clear later, but the functions that are called by each menu item must be defined in advance and cannot take arguments. If you want to call functions with dynamic inputs, use the PromptResponse class or the value of the active cell if you are using Google Spreadsheets. Menus can be modified in the sense that they can be replaced with an updated instance (copy) of the same menu. Any time the .createMenu()
is used to create a menu with a name that has already been used, the .addToUi()
method will then replace that original menu with the new menu.
Let’s Make a Custom Menu
Creating custom menus is very easy because the code that creates them exactly like the menu appears. Each chained method gets a new line to represent the menu feature that the method adds.
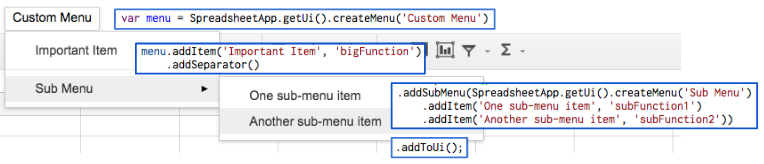
To create a menu that appears when the Google Spreadsheet or Google Docs application is opened, place the call to .createMenu()
inside the .onOpen
method.
function onOpen(e) { var menu = SpreadsheetApp.getUi().createMenu('My Custom Menu') menu.addItem('Important Item', 'bigFunction') .addSeparator() .addSubMenu(SpreadsheetApp.getUi().createMenu('Sub Menu') .addItem('One sub-menu item', 'subFunction1') .addItem('Another sub-menu item', 'subFunction2')) .addToUi(); // do not set a variable to any chain of methods ending in .addToUi() // after the Menu is created, the value of menu will be undefined // the .addToUi() method does not return anything. }
The Ui Class and the Menu Class
Custom menus make use of two Google Apps Script classes. The Ui Class offers the ability to add to and modify the user interface of the Google App that the script is bound to. This is everything from pop-up prompts and alerts to sidebars, to, of course, menus. The Ui class offers the only way to create a Menu object, with the .createMenu() method. The Menu class is much smaller in scope. The Menu class only offers the ability to define the appearance and functionality of a menu, and that is all. If you find yourself creating big menus with sub-menus, you might want to define each menu individually and pass them to their parent menu, in the .addSubMenu()
method. For illustration purposes:
var mainMenu = SpreadsheetApp.getUi().createMenu('Top Menu').addItem("Function 1", test); // Menu class object var subMenu = SpreadsheetApp.getUi().createMenu('Sub Menu').addItem("Function 2", test); // Menu class object var subSubMenu = SpreadsheetApp.getUi().createMenu('Sub Sub Menu').addItem("Function 3", test); // Menu class object subMenu.addSubMenu(subSubMenu) // passing in Menu object mainMenu.addSubMenu(subMenu) // passing in Menu object mainMenu.addToUi() // creates a 3 tier Menu (don't do that)
Three-tier menus are actually not a good idea. Here’s why:
Tips on User-Friendly Menus
At a minimum, menus should be easy to use and fast to load. For usability, Google’s Material UI Guidelines, recommends the following:
- Menu names and menu item names should be short, descriptive, and accurate, with sentence casing.
- Do not duplicate menu names or menu item names
- Menus should be nested no more than one level deep. (The example above was for example purposes.)
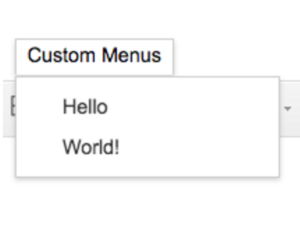
Get started! To see how these menus work. Copy and paste one of the scripts into the Google Apps Script editor and press play. I hope this helps keep the users of your custom Google App Script happy!